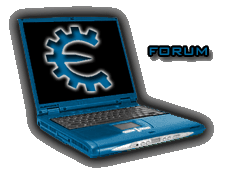 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
AfricanRainforest How do I cheat?
Reputation: 0
Joined: 10 Dec 2020 Posts: 4
|
Posted: Fri Mar 07, 2025 5:53 am Post subject: Hooking Direct3D9 and drawing shows nothing |
|
|
I've been trying to inject Scribblenauts and hooks it's d3d9 and draw my own stuff on top of the game.
I store it's Direct3DDevice9 instance through code injection and then hook EndScene(), the hook itself works fine and i can print out of the function. but no draw calls show any visual difference at all.
tried both drawing and clearing:
Code: | Vertex tri[] = {
{0.0f, 0.5f, 0.0f, D3DCOLOR_XRGB(255, 0, 0)},
{-0.5f, -0.5f, 0.0f, D3DCOLOR_XRGB(0, 255, 0)},
{0.5f, -0.5f, 0.0f, D3DCOLOR_XRGB(0, 0, 255)}
};
d3d_device->SetFVF(D3DFVF_XYZ | D3DFVF_DIFFUSE);
d3d_device->DrawPrimitiveUP(D3DPT_TRIANGLELIST, 1, tri, sizeof(Vertex));
LPDIRECT3DSURFACE9 backBuffer;
d3d_device->GetBackBuffer(0, 0, D3DBACKBUFFER_TYPE_MONO, &backBuffer);
d3d_device->SetRenderTarget(0, backBuffer);
d3d_device->Clear(0, nullptr, D3DCLEAR_TARGET, D3DCOLOR_XRGB(255, 0, 0), 1.0f, 0);
backBuffer->Release(); |
The only thing i know that shows any difference is setting it to render in wireframe mode:
Code: | d3d_device->SetRenderState(D3DRS_FILLMODE, D3DFILL_WIREFRAME); |
which turns the whole screen black where you only can see the game through the slits on it's edges and diagonal, suggesting the game renders to a quad and then draws that onto the screen.
either way, my draw calls are the last thing being done before EndScene() is called.
I also think EndScene() as well as every other d3d9 function is a dx12 function acting as dx9, but is shouldn't matter anyhow.
I've only worked with in OpenGL in the past, so most of the code is from ChatGPT, but this should show something atleast?
Description: |
|
Filesize: |
9.78 KB |
Viewed: |
8365 Time(s) |
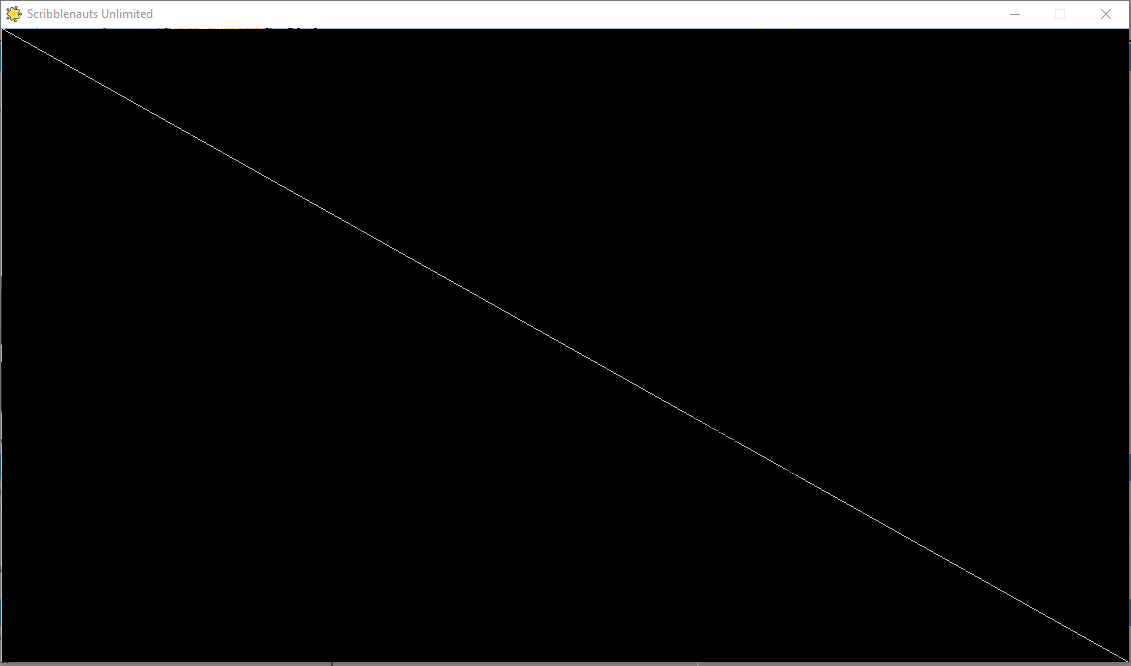
|
Description: |
|
Filesize: |
13.33 KB |
Viewed: |
8365 Time(s) |

|
|
|
Back to top |
|
 |
atom0s Moderator
Reputation: 204
Joined: 25 Jan 2006 Posts: 8577 Location: 127.0.0.1
|
Posted: Fri Mar 07, 2025 2:47 pm Post subject: |
|
|
Depending on what your end-goal is with what you plan to render, EndScene is generally not the most ideal place to hook and perform drawing inside of. This is because EndScene can be called multiple times per-frame causing your rendering code to run more than once when it doesn't need to be.
If your goal is to simply render things over the entire scene, then it is generally more ideal to hook IDirect3DDevice9::Present and perform your rendering there. (Do your rendering first, then allow the original call to Present to happen.)
Additionally, if you are not seeing things render properly, it is likely due to the current render state the device is in. You may need to do additional work first such as:
- Clearing any currently set textures that may interfere with your rendering. (via SetTexture)
- Clearing the currently set viewport which may be clipping your rendering. (via SetViewport)
- Properly setting up the current transforms. (via SetTransform)
- Properly setting up the various render states. (via SetRenderState)
- Properly setting up the texture stages (if needed). (via SetTextureStageState)
- Removing the currently set pixel shader. (via SetPixelShader)
- Removing the currently set vertex shader. (via SetVertexShader)
- etc.
If you do see that the render states need to be adjusted every time you plan to render, then you should also look into using a state block to both, preserve and restore the games own state, as well as to setup and set yours when needed.
Keep in mind, the manner in which you need to cleanup the current state of the device before/after rendering will vary by game. It may be required that you backup everything you are altering and restore it after you render in order for the game to function properly still. You can do that by using the various 'Get' variants of the same calls I listed above to backup the given information, apply your own changes, do your rendering, then restore the original values you backed up afterward.
_________________
- Retired. |
|
Back to top |
|
 |
AfricanRainforest How do I cheat?
Reputation: 0
Joined: 10 Dec 2020 Posts: 4
|
Posted: Tue Mar 11, 2025 11:49 am Post subject: |
|
|
Ok, I hooked present as and tried clearing all the states that could mess with rendering as you said:
Code: | printf("Hello from Present()\n");
d3d_device->BeginScene();
D3DVIEWPORT9 view;
d3d_device->GetViewport(&view);
printf("x: %i, y: %i, w: %i, h:%i\n", view.X, view.Y, view.Width, view.Height);
//viewport
D3DVIEWPORT9 vp = { 0, 0, view.Width, view.Height, 0.0f, 1.0f };
d3d_device->SetViewport(&vp);
//projection matrix
D3DMATRIX ortho = {
2.0f / view.X, 0, 0, 0,
0, -2.0f / view.Y, 0, 0,
0, 0, 1.0f, 0,
-1.0f, 1.0f, 0, 1.0f
};
d3d_device->SetTransform(D3DTS_PROJECTION, &ortho);
d3d_device->SetTransform(D3DTS_PROJECTION, &ortho);
//identity matrices
D3DMATRIX identity = {
1, 0, 0, 0,
0, 1, 0, 0,
0, 0, 1, 0,
0, 0, 0, 1
};
d3d_device->SetTransform(D3DTS_WORLD, &identity);
d3d_device->SetTransform(D3DTS_VIEW, &identity);
//render states
d3d_device->SetRenderState(D3DRS_ZENABLE, FALSE);
d3d_device->SetRenderState(D3DRS_ALPHABLENDENABLE, TRUE);
d3d_device->SetRenderState(D3DRS_SRCBLEND, D3DBLEND_SRCALPHA);
d3d_device->SetRenderState(D3DRS_DESTBLEND, D3DBLEND_INVSRCALPHA);
d3d_device->SetRenderState(D3DRS_LIGHTING, FALSE);
d3d_device->SetPixelShader(nullptr);
d3d_device->SetVertexShader(nullptr);
// Clear the screen
d3d_device->Clear(0, nullptr, D3DCLEAR_TARGET, D3DCOLOR_XRGB(255, 0, 0), 1.0f, 0);
d3d_device->EndScene(); |
the messages print, but the game looks no different.
i've tried everything i can think of at this point
i can also add that using CE's direct3D hook doesn't work either, i used it to try and put a crosshair on the screen with no success.
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|