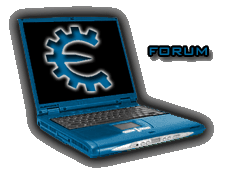 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
sreeragh2009 Cheater
Reputation: 0
Joined: 21 Oct 2014 Posts: 33 Location: UNKNOWN
|
Posted: Sat Mar 07, 2015 9:11 am Post subject: Using opcodes in C++ |
|
|
Just for info, i am a beginner and all i ever did in my life in coding is a calculator, now i am using c++ for game hacking but i got a problem. I got a opcode in game for example
Just an example* , now i got the memory address of this opcode , its software + 9A342 or something like that. Now that "eax" always is the address i want, and keeps on changing, so how do i implement in c++ ? How do i store the eax value ? Is there a function or something like that ?
_________________
PROFESSIONAL "LEARNER" |
|
Back to top |
|
 |
justa_dude Grandmaster Cheater
Reputation: 23
Joined: 29 Jun 2010 Posts: 891
|
Posted: Sat Mar 07, 2015 12:18 pm Post subject: |
|
|
Can you make the cheat in Cheat Engine? If you can't, I'm afraid that you will not be able to make it in C.
If you can make the cheat in CE, then I recommend you get the ASMJIT library and emulate CE using it. That is, write your ASM for injection using the meta-language ASMJIT provides (it's very similar to CE's AutoAssembly), then ask ASMJIT to assemble your code into the remote process. You will also have to inject a patch to jump to your code cave. Petr has reworked the library quite a bit since I wrote the following snippet, but the premise is the same:
Code: |
winmain()
...
invalidaterect = GetProcAddress(me32.hModule,"InvalidateRect");
updatewindow = GetProcAddress(me32.hModule,"UpdateWindow");
...
//some assembly code to turn all hidden bombs into flags
AssemblerEx a;
Label loop, not_bomb; //labels for utility
a.pushad(); //save regs
a.pushfd(); //save flags
a.mov(ptr_abs(reinterpret_cast<void *>(0x100579c)), edi); //reset timer to zero (original code)
a.mov(eax, imm(0x1005361)); //minefield address
a.mov(ecx, ptr_abs(reinterpret_cast<void *>(0x1005338))); //move num_rows into ecx
a.shl(ecx, 5); //each row is padded to 32 bytes, multiply ecx(num_rows) by 32 to get grid size
a.add(ecx, eax); //ecx should now point to the end of the minefield
a.bind(&loop); //loop:
a.cmp(byte_ptr(eax), imm(0x8f)); //is this position a bomb?
a.jne(¬_bomb); //jump if not a bomb
a.mov(byte_ptr(eax), imm(0x8e)); //this is a bomb, mark it with a flag
a.bind(¬_bomb); //not_bomb:
a.inc(eax); //advance eax to look at next position in grid
a.cmp(eax, ecx); //are we at the end of the grid?
a.jne(&loop); //if not, rinse and repeat
a.push(imm(1)); //all WINAPI functions use stdcall - push arguments onto the stack
a.push(imm(0)); //to call Windows API functions from Minesweeper's IAT
a.push(imm(reinterpret_cast<DWORD>(hwnd))); //recall hwnd to app & push it
a.call(invalidaterect); //call InvalidateRect to instruct Windows to repaint everything on next update
a.push(imm(reinterpret_cast<DWORD>(hwnd))); //push hwnd again for next API call
a.call(updatewindow); //call UpdateWindow to instruct Windows to send WM_PAINT to Minesweeper
a.popfd(); //restore flags
a.popad(); //restore regs
a.ret(); //return
void * code_cave = a.rmake(hproc); //assemble the code and store it in Minesweeper's address space
//some assembly to patch the game to jump to our code when starting a new game
AssemblerEx b;
b.call(code_cave);
b.nop(); //because instruction we're replacing is six bytes and our call instruction is only five
b.rmake(hproc, reinterpret_cast<void *>(0x0100371A));
return EXIT_SUCCESS;
}
|
This was a simple proof of concept trainer for Minesweeper that revealed all the bombs. If you're comfortable w/ CE and CPP, it will probably be obvious. To me, it appears strikingly elegant.
_________________
A nagy kapu mellett, mindig van egy kis kapu.
----------------------
Come on... |
|
Back to top |
|
 |
sreeragh2009 Cheater
Reputation: 0
Joined: 21 Oct 2014 Posts: 33 Location: UNKNOWN
|
Posted: Sat Mar 07, 2015 2:48 pm Post subject: |
|
|
Actually my problem is not using asm function inside c++, just to be straightforward i want to save a register at a paticular address ( software + offset opcode ) into a variable in c++. So i just want my code to grab the opcode, read the register, store the value to my variable. or maybe what i need is a pointer which points to my address but pointer scans dont go well on my pc , its just too slow and take too much size. anyway to use my opcode to get a pointer. Cause the opcode only acceses the address i need. thanks for the quick reply tho. soorry for spelling mistakes, typed it on mobile.
_________________
PROFESSIONAL "LEARNER" |
|
Back to top |
|
 |
justa_dude Grandmaster Cheater
Reputation: 23
Joined: 29 Jun 2010 Posts: 891
|
Posted: Sat Mar 07, 2015 3:13 pm Post subject: |
|
|
sreeragh2009 wrote: | Actually my problem is not using asm function inside c++ |
Any inline assembly you write is going to be statically compiled into your own executable. That's not going to help you patch the code of another process.
sreeragh2009 wrote: | just to be straightforward i want to save a register at a paticular address ( software + offset opcode ) into a variable in c++. |
Then you either need to patch the other process to store the pointer somewhere or implement your own debugger. Since you're probably trying to make a trainer rather than a full-on debugger, the former is your easiest route.
sreeragh2009 wrote: | or maybe what i need is a pointer which points to my address |
This would definitely simplify things from the C side, but that's not what you asked for and not something that you're going to solve easier with C than with Cheat Engine.
I maintain: if you can't make your cheat in Cheat Engine then you are not capable of making it in C.
Good luck,
JD
_________________
A nagy kapu mellett, mindig van egy kis kapu.
----------------------
Come on... |
|
Back to top |
|
 |
sreeragh2009 Cheater
Reputation: 0
Joined: 21 Oct 2014 Posts: 33 Location: UNKNOWN
|
Posted: Sun Mar 08, 2015 12:21 am Post subject: |
|
|
I made the full trainer using Auto assembler in cheat engine. But there we replace the opcode i need ,to jump to newmem, do my stuff and jump back. So you are saying there is no easy way to save a register into a variable.Ill try my luck with pointers. Ok so ill move on to next question, What is a hook ? Thaks for the fast reply.
_________________
PROFESSIONAL "LEARNER" |
|
Back to top |
|
 |
justa_dude Grandmaster Cheater
Reputation: 23
Joined: 29 Jun 2010 Posts: 891
|
Posted: Sun Mar 08, 2015 10:32 am Post subject: |
|
|
sreeragh2009 wrote: | I made the full trainer using Auto assembler in cheat engine. But there we replace the opcode i need ,to jump to newmem, do my stuff and jump back. |
Yes, that's why I showed you an example fragment that does just that.
sreeragh2009 wrote: | So you are saying there is no easy way to save a register into a variable. |
No, I'm saying that it's generally easier to make a trainer than a debugger. It's easy to save a register into a variable in C (it's built into the language), but it's much more complicated to read a register in another process at a specific time (it's not built into the language).
If you Google your own question (why didn't you start by doing that, btw?), you can find a thread on Stack Exchange asking the exact same question and providing the minimum necessary code to set a breakpoint and read a register. Coincidentally, the same page has Raymond Chen - notable hacker from Microsoft - responding that it's better to inject code than set a breakpoint.
Really, given all the options that've been explained/spoon-fed to you, there shouldn't be much left to say.
_________________
A nagy kapu mellett, mindig van egy kis kapu.
----------------------
Come on... |
|
Back to top |
|
 |
sreeragh2009 Cheater
Reputation: 0
Joined: 21 Oct 2014 Posts: 33 Location: UNKNOWN
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|