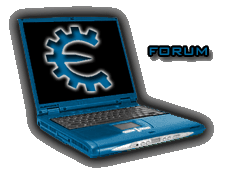 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
Turok2 How do I cheat?
Reputation: 0
Joined: 16 Feb 2024 Posts: 8
|
Posted: Tue Mar 19, 2024 11:46 pm Post subject: Key tsunami |
|
|
Hey buddy I've got this script that sets a software breakpoint, triggering the M key upon activation. The issue is that the game's animation is overly lengthy, causing the M key to be pressed around 50 times upon breakpoint activation. I need it to be pressed at most 3 times. I'm looking to initiate the start of the animation or resolve it differently, such as removing the breakpoint and auto-reactivating it, or suppressing the M key after it's been pressed 3 times. I'm open to trying different strategies.
local breakpointAddress = "Game.exe+123450"
local breakpointSet = false
local function onBreakpointHit()
keyDown(0x4D)
sleep(1)
keyUp(0x4D)
end
if not breakpointSet then
debug_setBreakpoint(breakpointAddress, onBreakpointHit)
breakpointSet = true
end
|
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 34
Joined: 16 Feb 2017 Posts: 1418
|
Posted: Wed Mar 20, 2024 5:19 am Post subject: |
|
|
Test this code in a separate script and see what it does.
When clicked 3 times (F8 key), it will aim to press and release the key.
After clicking 3 times (on the 4th click) it will aim to hold the key down 46 times and finally release it.
You can edit the number of prints, print sleep and hotkey.
Code: |
local breakpointIndx = 1
function onBreakpointStop()
--keyDown(0x4D)
print("Stop - breakpointIndx: "..breakpointIndx)
end
function onBreakpointHit()
if breakpointIndx<4 then -- stop limit 3
--keyDown(0x4D)
print(1,"keyDown(0x4D): "..breakpointIndx)
end
breakpointIndx = tonumber(breakpointIndx) + 1
if breakpointIndx>4 then -- start limit 4 + .. 46
for i=1, 46 do
sleep(10)
if breakpointIndx==50 then -- final func..
onBreakpointStop()
else
breakpointIndx = tonumber(breakpointIndx) + 1
--keyDown(0x4D)
print(2,"keyDown(0x4D): "..breakpointIndx) -- 4..50 press
end
end
end
sleep(10)
--keyUp(0x4D)
print(0,"keyUp(0x4D)\n")
end
if kyd1 then kyd1.Destroy() kyd1=nil end
kyd1=createHotkey(onBreakpointHit,VK_F8) |
_________________
|
|
Back to top |
|
 |
Turok2 How do I cheat?
Reputation: 0
Joined: 16 Feb 2024 Posts: 8
|
Posted: Wed Mar 20, 2024 11:27 am Post subject: |
|
|
AylinCE wrote: | Test this code in a separate script and see what it does.
When clicked 3 times (F8 key), it will aim to press and release the key.
After clicking 3 times (on the 4th click) it will aim to hold the key down 46 times and finally release it.
You can edit the number of prints, print sleep and hotkey.
Code: |
local breakpointIndx = 1
function onBreakpointStop()
--keyDown(0x4D)
print("Stop - breakpointIndx: "..breakpointIndx)
end
function onBreakpointHit()
if breakpointIndx<4 then -- stop limit 3
--keyDown(0x4D)
print(1,"keyDown(0x4D): "..breakpointIndx)
end
breakpointIndx = tonumber(breakpointIndx) + 1
if breakpointIndx>4 then -- start limit 4 + .. 46
for i=1, 46 do
sleep(10)
if breakpointIndx==50 then -- final func..
onBreakpointStop()
else
breakpointIndx = tonumber(breakpointIndx) + 1
--keyDown(0x4D)
print(2,"keyDown(0x4D): "..breakpointIndx) -- 4..50 press
end
end
end
sleep(10)
--keyUp(0x4D)
print(0,"keyUp(0x4D)\n")
end
if kyd1 then kyd1.Destroy() kyd1=nil end
kyd1=createHotkey(onBreakpointHit,VK_F8) |
|
Unfortunately, the method isn't working because the breakpoint keeps getting hit all the time, causing stuttering. However, I've changed the approach, and it seems to be working:
local breakpointAddress = "GAME.exe+123450"
local function onBreakpointHit()
debug_removeBreakpoint(breakpointAddress)
for i = 1, 3 do
keyDown(0x4D)
sleep(1)
keyUp(0x4D)
end
end
debug_setBreakpoint(breakpointAddress, onBreakpointHit)
This method sets a breakpoint on the address, then removes it as soon as it's hit. This process allows for the 3 "M" key presses in time, and afterward, by detaching itself, it doesn't disturb the game's performance. However, the issue is that I can't get it to reactivate on its own. If it could do so, say, after 1 second from pressing the "M" keys, the script would be perfect. I can't figure out how to do it. Thanks, as always, for your help!
|
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 34
Joined: 16 Feb 2017 Posts: 1418
|
Posted: Wed Mar 20, 2024 12:46 pm Post subject: |
|
|
Code: | if kydTim1 then kydTim1.Destroy() kydTim1=nil end
kydTim1 = createTimer(MainForm)
kydTim1.Interval=100
kydTim1.Enabled=false
mSec = 0
local breakpointAddress = "GAME.exe+123450"
local function onBreakpointHit()
debug_removeBreakpoint(breakpointAddress)
for i = 1, 3 do
keyDown(0x4D)
sleep(1)
keyUp(0x4D)
end
end
kydTim1.OnTimer=function()
if isKeyPressed(VK_M) then
for i=1, 10 do -- or sleep(1000) .. no mSec ..
mSec = tonumber(mSec) + 1
if mSec==10 then -- Interval(100) x 10 = 1 sec
debug_setBreakpoint(breakpointAddress, onBreakpointHit)
--print(2,"mSec: "..mSec)
mSec = 0
end
end
end
end
kydTim1.Enabled=true |
_________________
|
|
Back to top |
|
 |
Turok2 How do I cheat?
Reputation: 0
Joined: 16 Feb 2024 Posts: 8
|
Posted: Wed Mar 20, 2024 6:03 pm Post subject: |
|
|
AylinCE wrote: | Code: | if kydTim1 then kydTim1.Destroy() kydTim1=nil end
kydTim1 = createTimer(MainForm)
kydTim1.Interval=100
kydTim1.Enabled=false
mSec = 0
local breakpointAddress = "GAME.exe+123450"
local function onBreakpointHit()
debug_removeBreakpoint(breakpointAddress)
for i = 1, 3 do
keyDown(0x4D)
sleep(1)
keyUp(0x4D)
end
end
kydTim1.OnTimer=function()
if isKeyPressed(VK_M) then
for i=1, 10 do -- or sleep(1000) .. no mSec ..
mSec = tonumber(mSec) + 1
if mSec==10 then -- Interval(100) x 10 = 1 sec
debug_setBreakpoint(breakpointAddress, onBreakpointHit)
--print(2,"mSec: "..mSec)
mSec = 0
end
end
end
end
kydTim1.Enabled=true |
|
Unfortunately, I've tried, but when I activate this script, it doesn't set the breakpoint, and I don't understand why... Thank you for your help.
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|