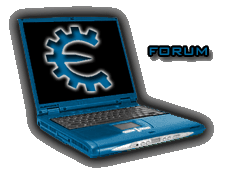 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
HZcjjNF How do I cheat?
Reputation: 0
Joined: 11 Aug 2023 Posts: 2
|
Posted: Fri Aug 11, 2023 10:11 am Post subject: [Solved] Scan foundlist yielding zero results |
|
|
Hi,
This is my first time creating a Lua script. What I'm trying to do is to automate a scan in my cheat table that tries to find a specific address that ends in "78" (e.g., 20E4F819E78). Pointer scan cannot be used since the address is within an emulator's virtual memory (Bluestacks Android Emulator) and it is allocated randomly every time.
This is what I've came up so far:
Code: |
{$lua}
[ENABLE]
local searchValue = inputQuery('Value Prompt', "HP: ",''); -- prompts the user for the initial HP value
local scan = createMemScan(true);
scan.setOnlyOneResult(false);
scan.firstScan(soExactValue, vtDword, rtRounded, searchValue, nil , 0 , 0xffffffffffffffff , "+W" , fsmLastDigits, 78 ,false ,false, false, false);
scan.waitTillDone();
local foundList = createFoundList(scan);
foundList.initialize();
print(foundList.Count); -- ALWAYS RETURNS 0
-- prompts the user for the current HP value if there are more than 1 results
while (foundList.Count > 1) do
searchValue = inputQuery('Value Prompt', "Current HP: ",'');
scan.nextScan(soExactValue, nil, searchValue, nil, false, false, false, false, false, "NEXT");
scan.waitTillDone();
foundList = createFoundList(scan);
end
-- Change HP value to 500000 once the address has been found (not working)
writeInteger(foundList.Address[0], 500000);
-- Cleanup
foundList.deinitialize();
foundList.destroy();
foundList = nil;
scan.destroy();
scan = nil;
[DISABLE]
|
The problem is that the foundList is always empty. Doing the scan manually on cheat engine does not yield the same result of course. Also, doing the manual scan seems to make the game freeze up a little bit, but the same thing does not happen when I'm running the script. What am I doing wrong?
Thanks in advance.
Last edited by HZcjjNF on Fri Aug 11, 2023 9:06 pm; edited 1 time in total |
|
Back to top |
|
 |
ParkourPenguin I post too much
Reputation: 140
Joined: 06 Jul 2014 Posts: 4309
|
Posted: Fri Aug 11, 2023 10:35 am Post subject: |
|
|
"alignmentparam" is a string, not a number. Use '78' instead. Even if numbers worked, they'd probably be in hex anyway (0x78).
I'd use an empty string instead of nil for the second input parameter.
Do NOT index a foundlist without checking if it's valid to do so first. Check if the count is 0 beforehand:
Code: | assert(foundList.Count > 0, 'No results found')
writeInteger(foundList.Address[0], 500000) |
Semicolons are unnecessary in Lua.
_________________
I don't know where I'm going, but I'll figure it out when I get there. |
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 32
Joined: 16 Feb 2017 Posts: 1264
|
Posted: Fri Aug 11, 2023 11:16 am Post subject: |
|
|
Try this.
Make sure that the "HP" value has changed before the second input to ensure you find the correct address during the scan.
Do the first scan, return to the game, increase or decrease HP, write the next HP value in the second scan.
Code: | [ENABLE]
{$lua}
local foundTbl = {}
searchValue = inputQuery('Value Prompt', "HP: ",''); -- prompts the user for the initial HP value
scan = createMemScan();
--scan.setOnlyOneResult(false);
scan.firstScan(soExactValue, vtDword, rtRounded, searchValue, "+W" , 0 , 0xffffffffffffffff , "" , fsmNotAligned, "1" ,false ,false, false, false);
scan.waitTillDone();
foundList = createFoundList(scan);
foundList.initialize();
print(1,foundList.Count); -- ALWAYS RETURNS 0
if foundList.Count > 0 then
for i=0, foundList.Count -1 do
res1 = (foundList.Address[i])
res = (res1):sub(-2,-1)
--print(res)
if res=="78" then
--print(foundList.Address[i])
foundTbl[#foundTbl + 1] = foundList.Address[i]
end
end
end
print(" ")
searchValue = inputQuery('Value Prompt', "Current HP: ",'');
for i,k in pairs(foundTbl) do
res3 = readInteger(k)
--print(res3)
if res3==tonumber(searchValue) then
--print(i,k)
writeInteger(k, 500000);
end
end
-- Cleanup
foundList.deinitialize();
foundList.destroy();
foundList = nil;
scan.destroy();
scan = nil;
{$asm}
[DISABLE] |
_________________
|
|
Back to top |
|
 |
HZcjjNF How do I cheat?
Reputation: 0
Joined: 11 Aug 2023 Posts: 2
|
Posted: Fri Aug 11, 2023 6:34 pm Post subject: |
|
|
I tried AylinCE's code and it is working perfectly. However, I want to learn more about cheat engine scripting so I tried to create one based from my original code and ParkourPenguin's answer.
The first scan is now working and no longer yielding zero results. However, the next scan is now the one that yields zero results.
EDIT: nevermind, I did a bit of experimentation and I came up with this one which works perfectly:
Code: |
[ENABLE]
{$lua}
searchValue = inputQuery('Value Prompt', "HP: ",''); -- prompts the user for the initial HP value
scan = createMemScan(true)
scan.setOnlyOneResult(false)
scan.firstScan(soExactValue, vtDword, rtRounded, searchValue, "" , 0 , 0xffffffffffffffff , "" , fsmLastDigits, "78" ,false ,false, false, false)
scan.waitTillDone()
foundList = createFoundList(scan)
foundList.initialize()
print(foundList.Count) -- usually yields more than 1 result
-- prompts the user for the current HP value if there are more than 1 result
while (foundList.Count > 1 and searchValue ~= "stop") do
-- After changing the value in-game
foundList.deinitialize()
searchValue = inputQuery('Value Prompt', "Current HP: ",'')
scan.nextScan(soExactValue, rtRounded, searchValue, "", false, false, false, false, false)
scan.waitTillDone()
foundList.initialize()
print(foundList.Count)
end
-- Change HP value to 500000 only if the address has been specified
if scan.FoundList.Count == 1 then
writeInteger(scan.FoundList.Address[0], 500000)
end
scan.destroy()
scan = nil
{$asm}
[DISABLE]
|
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|