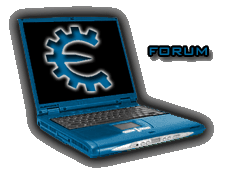 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
Mal1t1a Cheater
Reputation: 0
Joined: 03 Jan 2010 Posts: 40
|
Posted: Sun May 26, 2013 8:59 pm Post subject: Reliably call a function on memory change. |
|
|
Hello,
I'm trying to create a program that will allow me to call a function that I've programmed to alter the memory of the program in a specific manner.
My current application reads the memory using the "ReadProcessMemory" api. It stores the value of the location to a variable. It will then check the last value of the variable with the current value of the variable, if they are different it calls a function passing the variable as a parameter.
All of that works fine and dandy and does what I wanted it to do. However, because I don't know how to reliably read the memory location, sometimes the memory does change, but the application doesn't know.
I've read a bit online about something called a "code cave". I think I need to do this but I don't know. Also if I do, is there a simple tutorial that will show me how?
P.s. I usually do my work in vb.net, I know some C# and some basic C++ but vb.net has always done everything that I've needed, because I've only needed to write to the memory at a certain address that I can defeat DMA with using the read and write process memory apis. Which I guess writing a code cave is just recording the bytes, allocating some memory, and storing your function in the allocated memory location, and have it called by adding a jump to the newly allocated memory, then heading back to the program's loop by jumping back to the next bit of code to be called.
Can someone help me out with this?
|
|
Back to top |
|
 |
jucce Advanced Cheater
Reputation: 1
Joined: 02 Apr 2013 Posts: 99
|
Posted: Mon May 27, 2013 2:45 pm Post subject: |
|
|
Mal1t1a wrote: | All of that works fine and dandy and does what I wanted it to do. However, because I don't know how to reliably read the memory location, sometimes the memory does change, but the application doesn't know. | Do you mean that you want to monitor a specific memory address for changes? In that case what you can do is create a timer that triggers each second and checks the value for changes.
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Tue May 28, 2013 5:54 pm Post subject: |
|
|
If my assumption is correct, Mal1t1a already has a timer that periodically reads his target's memory, but sometimes his variable changes several times between each reads.
So how to "Reliably call a function on memory change"? Well the easiest way I know consist in turning your program into a dll, injecting it into the game, and hooking every function that writes your variable so that they call a function of that dll. That function will tell your program that the variable changed, and provide the new value.
Problem is that if the function is shared (ex: updates the player's position, but also those of the ennemies) you'll have to sort things out before calling or not MyDll.dll.UpdateMyVariable.
Anyway the approach above is done that way (sorting player vs enemies excluded):
1-Turn your program into a .dll.
2-In your dll export a function that will take the value of the thing you want to monitor as a parameter. In C it would be something like: Code: | __declspec( dllexport ) stdcall void UpdateMyVariable(const int NewValue); | 3-Stick cheat engine to your game, find your variable, then "find out what writes on this address".
4-Each line in the "find out what writes..." window will roughly look like "mov [eax],ebx". Click on it->show disassembler, then tools->autoassemble->template->cheat table framework.
5-Make sure "mov [eax],ebx" is selected in the disassembler then click then template->code injection.
6-In that pile of autogenerate code, locate your "mov [eax],ebx" and write: Code: | push ebx
call UpdateMyVariable //or MyDll.dll.UpdateMyVariable | just under.
7-Repeat steps 5-6 for each line in the "find out what writes..." window.
8-At the beginning of in the [enable] section, add 'loadlibrary("MyDll.dll")'. If CE complains about not understanding what UpdateMyVariable means, you need to make a separate script to load your dll, something like: Code: | [enable]
loadlibrary("MyDll.dll")
[disable] | and activate that script before validating the script that uses UpdateMyVariable.
9-Well if cheat engine agrees to add the big script to your cheat table, it should be good.
Apparently it is possible to write dlls in visual basic, but since I don't know how to call a function from a vb dll in assembly, I'd rewrite your program in C/C++.
|
|
Back to top |
|
 |
Pingo Grandmaster Cheater
Reputation: 8
Joined: 12 Jul 2007 Posts: 571
|
Posted: Wed May 29, 2013 3:06 am Post subject: |
|
|
Gniarf
Thats the method i usually use aswell but never in a dll.
I hook the function in C# or VB and store the single or multiple addresses.
You can write managed dlls and inject them using a bootstrap dll or loading it directly from memory. My injector uses the memory method.
Mal1t1a
See if you can find a static pointer first (easiest).
If thats not possible, you'l need to use code injection and store
the register (hardest but better).
_________________
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Thu May 30, 2013 2:35 pm Post subject: |
|
|
Pingo wrote: | You can write managed dlls and inject them using a bootstrap dll | Ah yes, a proxy dll, hadn't thought about it. I'll keep that in a corner of my memory, thanks!
@Mal1t1a: Thinking again about it, there is a method that is at least equally simple to reliably call a function on memory change: using hardware breakpoints. The idea is write and inject a dll, set up us the bomb *ahem* the breakpoint when DllMain(...) is called, and when the breakpoint is hit do whatever you want to do when the variable changes.
Setting a breakpoint is easy, there are tons of sample sources codes available on the net. (I use this one)
Handling what to do when the break is hit is done that way:
Code: |
HWHooker TheHook;
PVOID ExceptionHandle;
LONG CALLBACK ExceptionHandler(PEXCEPTION_POINTERS ExceptionInfo)
{
if (ExceptionInfo->ExceptionRecord->ExceptionAddress==reinterpret_cast<PVOID>(MONITORED_ADDRESS)&&
ExceptionInfo->ExceptionRecord->ExceptionCode==EXCEPTION_SINGLE_STEP)
{ //if it's a breakpoint at MONITORED_ADDRESS...
//do what you want with MONITORED_ADDRESS
//......
//job done, go play!
return EXCEPTION_CONTINUE_EXECUTION;
}
//wrong exception
return EXCEPTION_CONTINUE_SEARCH;
}
BOOL APIENTRY DllMain( HMODULE hModule, DWORD ul_reason_for_call,LPVOID lpReserved)
{
switch (ul_reason_for_call)
{
case DLL_PROCESS_ATTACH:
{
ExceptionHandle=AddVectoredExceptionHandler(1337,ExceptionHandler);
//ExceptionHandler is the function that will be called when ANY breakpoint or ANY other exception occurs
//set up your breakpoint here
TheHook.SetBreakpoint(HWHooker::WRITE,VARIABLE_SIZE,reinterpret_cast<void*>(MONITORED_ADDRESS));
break;
}
case DLL_THREAD_ATTACH:
case DLL_THREAD_DETACH:
case DLL_PROCESS_DETACH:
break;
}
return TRUE;
} |
The problem with this approach is that:
-Games with any decent DRM or anti-cheat protections will crash on you for using breakpoints.
-If MONITORED_ADDRESS is written very often, it may cause a severe slowdown.
-When your variable gets reallocated, you have to manually update the breakpoint with the new address.
But on the other hand you don't have to worry about shared functions.
|
|
Back to top |
|
 |
Mal1t1a Cheater
Reputation: 0
Joined: 03 Jan 2010 Posts: 40
|
Posted: Fri May 31, 2013 6:33 pm Post subject: |
|
|
jucce wrote: | Mal1t1a wrote: | All of that works fine and dandy and does what I wanted it to do. However, because I don't know how to reliably read the memory location, sometimes the memory does change, but the application doesn't know. | Do you mean that you want to monitor a specific memory address for changes? In that case what you can do is create a timer that triggers each second and checks the value for changes. |
Yeah I did do that, it's not reliable.
Gniarf wrote: | If my assumption is correct, Mal1t1a already has a timer that periodically reads his target's memory, but sometimes his variable changes several times between each reads.
So how to "Reliably call a function on memory change"? Well the easiest way I know consist in turning your program into a dll, injecting it into the game, and hooking every function that writes your variable so that they call a function of that dll. That function will tell your program that the variable changed, and provide the new value.
Problem is that if the function is shared (ex: updates the player's position, but also those of the ennemies) you'll have to sort things out before calling or not MyDll.dll.UpdateMyVariable.
Anyway the approach above is done that way (sorting player vs enemies excluded):
1-Turn your program into a .dll.
2-In your dll export a function that will take the value of the thing you want to monitor as a parameter. In C it would be something like: Code: | __declspec( dllexport ) stdcall void UpdateMyVariable(const int NewValue); | 3-Stick cheat engine to your game, find your variable, then "find out what writes on this address".
4-Each line in the "find out what writes..." window will roughly look like "mov [eax],ebx". Click on it->show disassembler, then tools->autoassemble->template->cheat table framework.
5-Make sure "mov [eax],ebx" is selected in the disassembler then click then template->code injection.
6-In that pile of autogenerate code, locate your "mov [eax],ebx" and write: Code: | push ebx
call UpdateMyVariable //or MyDll.dll.UpdateMyVariable | just under.
7-Repeat steps 5-6 for each line in the "find out what writes..." window.
8-At the beginning of in the [enable] section, add 'loadlibrary("MyDll.dll")'. If CE complains about not understanding what UpdateMyVariable means, you need to make a separate script to load your dll, something like: Code: | [enable]
loadlibrary("MyDll.dll")
[disable] | and activate that script before validating the script that uses UpdateMyVariable.
9-Well if cheat engine agrees to add the big script to your cheat table, it should be good.
Apparently it is possible to write dlls in visual basic, but since I don't know how to call a function from a vb dll in assembly, I'd rewrite your program in C/C++. |
It sounds as though this method uses cheat engine as the injector for the dll?
And just so I can understand what is being done, I'm just pushing the value onto the memory stack and when I do "call updateMyVariable" it will pass the value that has been pushed as the parameter?
Yeah I'm not too good with libraries either, I just write a .vb file that contains my functions and add it to each project.
Pingo wrote: | Gniarf
Thats the method i usually use aswell but never in a dll.
I hook the function in C# or VB and store the single or multiple addresses.
You can write managed dlls and inject them using a bootstrap dll or loading it directly from memory. My injector uses the memory method.
Mal1t1a
See if you can find a static pointer first (easiest).
If thats not possible, you'l need to use code injection and store
the register (hardest but better). |
There are certain situations where I have been able to find the static address, but others where I have been unsuccessful.
I like the method that works the best because I need the reliability. If I knew how to inject code and store the register that would be very beneficial. My language of choice is vb, I can handle C# as a fallback. If it can't be done in either of those, then I'll take the gruesome task of learning how to achieve it in C++.
Gniarf wrote: | Pingo wrote: | You can write managed dlls and inject them using a bootstrap dll | Ah yes, a proxy dll, hadn't thought about it. I'll keep that in a corner of my memory, thanks!
@Mal1t1a: Thinking again about it, there is a method that is at least equally simple to reliably call a function on memory change: using hardware breakpoints. The idea is write and inject a dll, set up us the bomb *ahem* the breakpoint when DllMain(...) is called, and when the breakpoint is hit do whatever you want to do when the variable changes.
Setting a breakpoint is easy, there are tons of sample sources codes available on the net. (I use this one)
Handling what to do when the break is hit is done that way:
Code: |
HWHooker TheHook;
PVOID ExceptionHandle;
LONG CALLBACK ExceptionHandler(PEXCEPTION_POINTERS ExceptionInfo)
{
if (ExceptionInfo->ExceptionRecord->ExceptionAddress==reinterpret_cast<PVOID>(MONITORED_ADDRESS)&&
ExceptionInfo->ExceptionRecord->ExceptionCode==EXCEPTION_SINGLE_STEP)
{ //if it's a breakpoint at MONITORED_ADDRESS...
//do what you want with MONITORED_ADDRESS
//......
//job done, go play!
return EXCEPTION_CONTINUE_EXECUTION;
}
//wrong exception
return EXCEPTION_CONTINUE_SEARCH;
}
BOOL APIENTRY DllMain( HMODULE hModule, DWORD ul_reason_for_call,LPVOID lpReserved)
{
switch (ul_reason_for_call)
{
case DLL_PROCESS_ATTACH:
{
ExceptionHandle=AddVectoredExceptionHandler(1337,ExceptionHandler);
//ExceptionHandler is the function that will be called when ANY breakpoint or ANY other exception occurs
//set up your breakpoint here
TheHook.SetBreakpoint(HWHooker::WRITE,VARIABLE_SIZE,reinterpret_cast<void*>(MONITORED_ADDRESS));
break;
}
case DLL_THREAD_ATTACH:
case DLL_THREAD_DETACH:
case DLL_PROCESS_DETACH:
break;
}
return TRUE;
} |
The problem with this approach is that:
-Games with any decent DRM or anti-cheat protections will crash on you for using breakpoints.
-If MONITORED_ADDRESS is written very often, it may cause a severe slowdown.
-When your variable gets reallocated, you have to manually update the breakpoint with the new address.
But on the other hand you don't have to worry about shared functions. |
I do enjoy that there is code attached to this post, however I can not use breakpoints in the game as they crash the application as you have mentioned.
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Fri May 31, 2013 8:02 pm Post subject: |
|
|
Mal1t1a wrote: | Gniarf wrote: | Anyway the approach above is done that way (sorting player vs enemies excluded):
1-Turn your program into a .dll.
2-In your dll export a function that will take the value of the thing you want to monitor as a parameter. In C it would be something like: Code: | __declspec( dllexport ) stdcall void UpdateMyVariable(const int NewValue); | 3-Stick cheat engine to your game, find your variable, then "find out what writes on this address".
4-Each line in the "find out what writes..." window will roughly look like "mov [eax],ebx". Click on it->show disassembler, then tools->autoassemble->template->cheat table framework.
5-Make sure "mov [eax],ebx" is selected in the disassembler then click then template->code injection.
6-In that pile of autogenerate code, locate your "mov [eax],ebx" and write: Code: | push ebx
call UpdateMyVariable //or MyDll.dll.UpdateMyVariable | just under.
7-Repeat steps 5-6 for each line in the "find out what writes..." window.
8-At the beginning of in the [enable] section, add 'loadlibrary("MyDll.dll")'. If CE complains about not understanding what UpdateMyVariable means, you need to make a separate script to load your dll, something like: Code: | [enable]
loadlibrary("MyDll.dll")
[disable] | and activate that script before validating the script that uses UpdateMyVariable.
9-Well if cheat engine agrees to add the big script to your cheat table, it should be good. |
It sounds as though this method uses cheat engine as the injector for the dll? | Yes it does. Since it already uses CE to patch all functions that write to the monitored address, I used CE to inject the dll aswell.
Mal1t1a wrote: | And just so I can understand what is being done, I'm just pushing the value onto the memory stack and when I do "call updateMyVariable" it will pass the value that has been pushed as the parameter? | Exactly. Calling a C function declared as:
Code: | void stdcall Foo(int Parameter1,bool Parameter2,Something* Parameter3) | is done that way in assembly:
Code: | push Parameter3
push Parameter2
push Parameter1
call Foo | In 32bit applications. I heard it's more hairy to call vb functions, something to do with a CoCreateInstance (I'm not competent on this topic), so if you can't rewrite your vb code in C then you should probably do as Pingo hinted: make your code in a vb dll and make a minimal C dll that simply calls your vb code, but from C code. Then call your C code from assembly.
|
|
Back to top |
|
 |
Mal1t1a Cheater
Reputation: 0
Joined: 03 Jan 2010 Posts: 40
|
Posted: Fri May 31, 2013 8:37 pm Post subject: |
|
|
Gniarf wrote: | Mal1t1a wrote: | Gniarf wrote: | Anyway the approach above is done that way (sorting player vs enemies excluded):
1-Turn your program into a .dll.
2-In your dll export a function that will take the value of the thing you want to monitor as a parameter. In C it would be something like: Code: | __declspec( dllexport ) stdcall void UpdateMyVariable(const int NewValue); | 3-Stick cheat engine to your game, find your variable, then "find out what writes on this address".
4-Each line in the "find out what writes..." window will roughly look like "mov [eax],ebx". Click on it->show disassembler, then tools->autoassemble->template->cheat table framework.
5-Make sure "mov [eax],ebx" is selected in the disassembler then click then template->code injection.
6-In that pile of autogenerate code, locate your "mov [eax],ebx" and write: Code: | push ebx
call UpdateMyVariable //or MyDll.dll.UpdateMyVariable | just under.
7-Repeat steps 5-6 for each line in the "find out what writes..." window.
8-At the beginning of in the [enable] section, add 'loadlibrary("MyDll.dll")'. If CE complains about not understanding what UpdateMyVariable means, you need to make a separate script to load your dll, something like: Code: | [enable]
loadlibrary("MyDll.dll")
[disable] | and activate that script before validating the script that uses UpdateMyVariable.
9-Well if cheat engine agrees to add the big script to your cheat table, it should be good. |
It sounds as though this method uses cheat engine as the injector for the dll? | Yes it does. Since it already uses CE to patch all functions that write to the monitored address, I used CE to inject the dll aswell.
Mal1t1a wrote: | And just so I can understand what is being done, I'm just pushing the value onto the memory stack and when I do "call updateMyVariable" it will pass the value that has been pushed as the parameter? | Exactly. Calling a C function declared as:
Code: | void stdcall Foo(int Parameter1,bool Parameter2,Something* Parameter3) | is done that way in assembly:
Code: | push Parameter3
push Parameter2
push Parameter1
call Foo | In 32bit applications. I heard it's more hairy to call vb functions, something to do with a CoCreateInstance (I'm not competent on this topic), so if you can't rewrite your vb code in C then you should probably do as Pingo hinted: make your code in a vb dll and make a minimal C dll that simply calls your vb code, but from C code. Then call your C code from assembly. |
so if I had
Code: |
void Bar(int Foo)
{
if(foo mod 2 == 1)
{
foo -= 1
pop foo
}
}
|
I can type in assembly
and the code will work?
Also In regards to 32 bit applications, I'm on a 64 bit environment so that's not much of a problem to me. I'll search around for making vb dll files on the internet. I use readprocessmemory and writeprocessmemory, and I'm not sure if those are the write functions that I should be using??
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Fri May 31, 2013 9:44 pm Post subject: |
|
|
Mal1t1a wrote: | so if I had
Code: | void Bar(int Foo)
{
if(foo mod 2 == 1)
{
foo -= 1
pop foo
}
} |
| ...it wouldn't compile because "pop" doesn't exist in C, C is case sensitive, and "void" functions cannot output a result. All in all I assume you meant: Code: | int Bar(int Foo)
{
if(Foo % 2 == 1)
{
Foo -= 1;
}
return Foo;
} |
Mal1t1a wrote: | I can type in assembly
and the code will work? | Nope, CE will bash you because it doesn't know "var" and neither do I.
...If it's a typo and you meant "call Bar", yes, that would work.
|
|
Back to top |
|
 |
Mal1t1a Cheater
Reputation: 0
Joined: 03 Jan 2010 Posts: 40
|
Posted: Mon Jun 03, 2013 9:43 pm Post subject: |
|
|
Gniarf wrote: | Mal1t1a wrote: | so if I had
Code: | void Bar(int Foo)
{
if(foo mod 2 == 1)
{
foo -= 1
pop foo
}
} |
| ...it wouldn't compile because "pop" doesn't exist in C, C is case sensitive, and "void" functions cannot output a result. All in all I assume you meant: Code: | int Bar(int Foo)
{
if(Foo % 2 == 1)
{
Foo -= 1;
}
return Foo;
} |
Mal1t1a wrote: | I can type in assembly
and the code will work? | Nope, CE will bash you because it doesn't know "var" and neither do I.
...If it's a typo and you meant "call Bar", yes, that would work. |
oops, haha. I did mean to type in "Bar".
So if I wanted to push a string into a function would I do the same thing?
Code: |
string Barber(string Fools)
{
return (replace(Fools.begin(), Fools.end(), 'p', 'q'));
}
|
then in cheat engine assembly I just add the two lines:
Code: |
push Fools
Call Barber
|
also, if I have my .dll ready, where do I place it for cheat engine to inject it?
edit:
Okay so I have this code in vb.net as a library class (it compiles into MemoryTest.dll)
Code: |
Public Class MemoryTest
Public Function OnHealthChange(ByVal HP As Integer) As Integer
Return HP + 1
End Function
End Class
|
in cheat engine I get this as my calling code:
I go to show disassembler->tools->Auto Assemble->Template->Cheat Table relocation framework
I enter loadlibrary("TestMemory.dll")
Then I do the same thing except this time select: code injection
which compiles to this:
Code: |
alloc(newmem,2048) //2kb should be enough
label(returnhere)
label(originalcode)
label(exit)
newmem: //this is allocated memory, you have read,write,execute access
mov [edi],eax //this is the original code
push eax //this is the integer I want to push
call OnHealthChange //this is the function i want to call
mov eax,edi
pop edi//place your code here
originalcode:
mov [edi],eax
mov eax,edi
pop edi
exit:
jmp returnhere
"server.dll"+310CF:
jmp newmem
returnhere:
|
I can't run the code for the loadlibrary dll. It says it cannot inject the dll (where is it supposed to be?)
so then I went to the memory viewer, selected tools -> inject dll ->browsed for dll -> selected it -> "Do you want to run a function?" -> yes -> dll injection successful
Then I went to the auto assemble page, with the code that calls the function. I proceed to click execute. Then the game freezes and crashes.
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Mon Jun 03, 2013 11:37 pm Post subject: |
|
|
Mal1t1a wrote: | So if I wanted to push a string into a function would I do the same thing?
Code: |
string Barber(string Fools)
{
return (replace(Fools.begin(), Fools.end(), 'p', 'q'));
}
|
then in cheat engine assembly I just add the two lines:
Code: |
push Fools
Call Barber
|
| First "string" in C++ is a very high level class to handle a sequence of characters, and constructing a class object in assembly is more pain than needed. Instead it is easier to use the char basic type (or rather an array of char), like:
Code: | __declspec( dllexport ) stdcall char* Barber(char* Fools)
{
static char Output[256]; //static means that there will only be one instance of this buffer, so beware if you use Barber to process several strings.
CString EasyToManipulateString=Fools; //CString has an handier replace function than string
EasyToManipulateString.Replace('p','q');
strcpy(Output,EasyToManipulateString.GetBuffer());
return Output;
} |
Mal1t1a wrote: | also, if I have my .dll ready, where do I place it for cheat engine to inject it? | The folder that contains the game's exe? Alternatively you can write "loadlibrary("c:\\temp\\MyDll.dll")" (I don't remember if it's \ or \\ in AA scripts).
Mal1t1a wrote: | Okay so I have this code in vb.net as a library class (it compiles into MemoryTest.dll)
[...]
I enter loadlibrary("TestMemory.dll") | Is MemoryTest.dll and TestMemory.dll the same file, or is TestMemory a proxy dll in C that calls the vb one?
Mal1t1a wrote: | code injection which compiles to this:
[snip] | Tskk tskk tskk, you edited it and in doing so, added bugs. It should have been:
Code: |
alloc(newmem,2048) //2kb should be enough
label(returnhere)
label(originalcode)
label(exit)
newmem: //this is allocated memory, you have read,write,execute access
//nothing here
originalcode:
mov [edi],eax
push eax //this is the integer I want to push
call OnHealthChange //this is the function i want to call
mov eax,edi
pop edi
exit:
jmp returnhere
"server.dll"+310CF:
jmp newmem
returnhere:
|
newmem and originalcode are just names given to code addresses like game.exe=0x400000. They are not section delimiters. In the code below
Code: |
newmem:
add eax,1
originalcode:
mov [ebx],eax
jmp returnhere
Game.exe+124
jmp newmem
returnhere
| your cpu will see jmp newmem, then add eax,1, then it will proceed to the next instruction: mov [ebx],eax, and then jmp return here.
Note:in your script you can remove both lines mentioning originalcode ,and both lines mentioning "exit" since they are useless in your script.
Mal1t1a wrote: | Then I went to the auto assemble page, with the code that calls the function. I proceed to click execute. Then the game freezes and crashes. | Given your AA srcipt it had to happen, regardless of the content of your dll, but make sure the function you call from asm is an stdcall C function, not a VB one.
|
|
Back to top |
|
 |
Mal1t1a Cheater
Reputation: 0
Joined: 03 Jan 2010 Posts: 40
|
Posted: Tue Jun 04, 2013 3:57 pm Post subject: |
|
|
Okay so I will try approaching this differently.
I was using a .dll that was coded in VB.net.
Now I tried to use a C++ .dll file.
This is the code I have for the C++ .dll file
Code: |
// MemoryHookTest.cpp : Defines the exported functions for the DLL application.
//
#include "stdafx.h"
__declspec( dllexport ) int* __stdcall Barber(int* Fools)
{
*Fools += 1;
return Fools;
}
|
When I build it, it compiles into "MemoryHookTest.dll"
Here is a list of what I do to hack the application:
1. I find the value that I want to change.
2. I find the address that writes to the value.
3. I show disassembler
4. I click Tools->Inject DLL
5. I browse to the MemoryHookTest.dll file.
6. I click "No" to the "Do you want to execute a function?
7. I click Tools->Auto Assemble
8. I click Template->Inject Code
9. This is the code I am given:
Code: |
alloc(newmem,2048) //2kb should be enough
label(returnhere)
label(originalcode)
label(exit)
newmem: //this is allocated memory, you have read,write,execute access
//place your code here
originalcode:
mov [edx+00000148],eax
exit:
jmp returnhere
001C1643:
jmp newmem
nop
returnhere:
|
10. I add my two calls to the code:
Code: |
alloc(newmem,2048) //2kb should be enough
label(returnhere)
label(originalcode)
label(exit)
newmem: //this is allocated memory, you have read,write,execute access
//place your code here
originalcode:
mov [edx+00000148],eax
push eax
call Barber
exit:
jmp returnhere
001C1643:
jmp newmem
nop
returnhere:
|
11. Then I click Execute, it injects successfully.
12. Then I go back into the program, and force the value to change.
13. I watch as the application crashes.
14. I come on here to post everything I did step by step in hopes of getting an accurate answer.
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Tue Jun 04, 2013 4:57 pm Post subject: |
|
|
Mal1t1a wrote: | Now I tried to use a C++ .dll file. | As a dotNET hater I congratulate you!
Code: |
alloc(newmem,2048) //2kb should be enough
label(returnhere)
label(originalcode)
label(exit)
newmem: //this is allocated memory, you have read,write,execute access
//place your code here
originalcode:
mov [edx+00000148],eax
push eax
call Barber
exit:
jmp returnhere
001C1643:
jmp newmem
nop
returnhere:
| It's a detail for now, but make sure memory editor->view->show symbols is enabled. Normally 001C1643 should be shown as "Something.exe+12345". Anyway it's unrelated to your current problem.
Mal1t1a wrote: | 13. I watch as the application crashes. | Thought wtf until I built your dll, injected it, crashed a program, and stuck a debugger to it...then I understood.
In:
Code: | mov [edx+00000148],eax
push eax
call Barber | You're passing eax=the number of idiots as a parameter. However Barber(int* Fools) expects the ADRESSS OF the number of fools. So when there is one retard, the barber tries to read the number the number fools, which you told him was stored at address 0x1 -> access violation-> crash. To pass the address of Fools (which is edx+00000148) to Barber, replace push eax+call Barber by:
Code: | push eax //this one means "make a backup of eax" not "pass eax as argument"
lea eax, [edx+148] //now eax=edx+148
push eax //argument
call MemoryHookTest.Barber //better than Barber alone, incase another dll exports a function with the same name
pop eax //restore the backup made above |
|
|
Back to top |
|
 |
Mal1t1a Cheater
Reputation: 0
Joined: 03 Jan 2010 Posts: 40
|
Posted: Tue Jun 04, 2013 8:54 pm Post subject: |
|
|
Sweet!
It works!
Although, I'm too inexperienced in C++ to write what I want to do.
Should I make a separate topic about trying to call a function from a vb.net library in C++? I mean I think that's the only way that I would be able to write a memory hook that will call a vb.net function.
As far as this method of using a C++ only .dll file, how would I do the same thing, but for a string of unknown length.
The way I determined how long the string was is to read in 255 bytes, parse it until I hit the byte "00" and then concatenate everything before it.
I don't know if there is a better way.
|
|
Back to top |
|
 |
Gniarf Grandmaster Cheater Supreme
Reputation: 43
Joined: 12 Mar 2012 Posts: 1285
|
Posted: Tue Jun 04, 2013 9:20 pm Post subject: |
|
|
Mal1t1a wrote: | Although, I'm too inexperienced in C++ to write what I want to do. | May I inquire what you want to do?
Mal1t1a wrote: | Should I make a separate topic about trying to call a function from a vb.net library in C++? | I'd recommend googling instead, since someone MUST have had this issue before you. Otherwise the General programming should be appropriate.
Mal1t1a wrote: | As far as this method of using a C++ only .dll file, how would I do the same thing, but for a string of unknown length. | Err the "same thing"? Same as what?
Mal1t1a wrote: | The way I determined how long the string was is to read in 255 bytes, parse it until I hit the byte "00" and then concatenate everything before it. | strlen(AddressOfTheFirstCharOfAString); will give you the total length of that string.
Note: technically, strlen just searches for the first 00 in a string.
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|