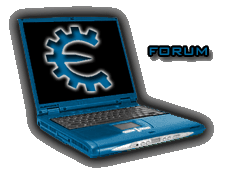 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
Dark Byte Site Admin
Reputation: 458
Joined: 09 May 2003 Posts: 25298 Location: The netherlands
|
Posted: Thu Jun 09, 2016 10:05 am Post subject: cpuid and affinity |
|
|
sometimes you may wish to call cpuid.
this script adds the CPUID(int) function
Code: |
autoAssemble([[
alloc(cpuidcaller, 128)
registersymbol(cpuidcaller)
cpuidcaller:
//parameter is a pointer to a block of 16 bytes which acts as input and output
//layout:
//index / eax_result
//ebx_result
//ecx_result
//edx_result
[32-bit]
mov eax,[esp+4]
mov eax,[eax]
push ebx
push ecx
push edx
[/32-bit]
[64-bit]
mov rax,[rcx]
mov r8,rcx //save rcx
mov r9,rbx //save rbx
[/64-bit]
cpuid
[32-bit]
push edi
mov edi,[esp+14]
mov [edi],eax
mov [edi+4],ebx
mov [edi+8],ecx
mov [edi+c],edx
pop edi
pop edx
pop ecx
pop ebx
ret 4
[/32-bit]
[64-bit]
mov [r8],eax
mov [r8+4],ebx
mov [r8+8],ecx
mov [r8+c],edx
mov rbx,r9 //restore rbx
ret
[/64-bit]
]], true)
function CPUID(i,j)
local s=createMemoryStream()
s.Size=20 --allocate 20 bytes (yes, you can do this)
writeIntegerLocal(s.Memory,i)
writeIntegerLocal(s.Memory+8,j)
executeCodeLocal("cpuidcaller", s.Memory)
local r={}
r.eax=readIntegerLocal(s.Memory)
r.ebx=readIntegerLocal(s.Memory+4)
r.ecx=readIntegerLocal(s.Memory+8)
r.edx=readIntegerLocal(s.Memory+12)
s.destroy()
return r
end
|
_________________
Do not ask me about online cheats. I don't know any and wont help finding them.
Like my help? Join me on Patreon so i can keep helping |
|
Back to top |
|
 |
Dark Byte Site Admin
Reputation: 458
Joined: 09 May 2003 Posts: 25298 Location: The netherlands
|
Posted: Fri Jun 17, 2016 5:35 am Post subject: |
|
|
And related, sometimes setting and getting the affinity of a process or thread could come in handy as well.
This code can be used to add GetAffinity, SetAffinity and SetAffinityThread
Code: |
autoAssemble([[
alloc(GetAffinity, 128)
registersymbol(GetAffinity)
GetAffinity:
[32-bit]
mov eax,[esp+4]
push [eax+8]
push [eax+4]
push ffffffff //push [eax]
[/32-bit]
[64-bit]
sub rsp,28
mov rax,rcx
mov rcx,ffffffffffffffff //[rax]
mov rdx,[rax+8]
mov r8,[rax+10]
[/64-bit]
call GetProcessAffinityMask
[32-bit]
ret 4
[/32-bit]
[64-bit]
add rsp,28
ret
[/64-bit]
]], true)
function GetAffinity(ph)
local psize
local s=createMemoryStream()
if cheatEngineIs64Bit() then
psize=8
s.Size=24
else
psize=4
s.Size=12
end
if ph==nil then
writePointerLocal(s.Memory,-1) --currentProcessHandle
else
writePointerLocal(s.Memory,ph)
end
writePointerLocal(s.Memory+psize,s.Memory)
writePointerLocal(s.Memory+psize*2,s.Memory+psize)
if executeCodeLocal("GetAffinity", s.Memory)==0 then return nil end
local r={}
r.ProcessAffinity=readPointerLocal(s.Memory)
r.SystemAffinity=readPointerLocal(s.Memory+psize);
s.destroy()
return r
end
----------------------------------------------------------------------
-- Set Affinity --
----------------------------------------------------------------------
autoAssemble([[
alloc(SetAffinity, 128)
registersymbol(SetAffinity)
SetAffinity:
[32-bit]
mov eax,[esp+4]
push [eax+4]
push [eax]
[/32-bit]
[64-bit]
sub rsp,28
mov rax,rcx
mov rcx,[rax]
mov rdx,[rax+8]
[/64-bit]
call SetProcessAffinityMask
[32-bit]
ret 4
[/32-bit]
[64-bit]
add rsp,28
ret
[/64-bit]
]], true)
function SetAffinity(mask, ph)
local psize
local s=createMemoryStream()
if cheatEngineIs64Bit() then
psize=8
s.Size=16
else
psize=4
s.Size=8
end
if ph==nil then
writePointerLocal(s.Memory,-1) --currentProcessHandle
else
writePointerLocal(s.Memory,ph)
end
writePointerLocal(s.Memory+psize,mask)
if executeCodeLocal("SetAffinity", s.Memory)==0 then return nil end
s.destroy()
return true
end
----
autoAssemble([[
alloc(SetAffinityThread, 128)
registersymbol(SetAffinityThread)
SetAffinityThread:
[32-bit]
mov eax,[esp+4]
push [eax+4]
push [eax]
//call GetCurrentThread
//push eax
[/32-bit]
[64-bit]
push rbx
push rdi
sub rsp,28
mov rbx,rcx
// call GetCurrentThread
// mov rcx,rax
mov rcx,[rbx]
mov rdx,[rbx+8]
[/64-bit]
//call SetThreadAffinityMask
[32-bit]
ret 4
[/32-bit]
[64-bit]
add rsp,28
pop rdi
pop rbx
ret
[/64-bit]
]], true)
function SetAffinityThread(mask, th)
local psize
local s=createMemoryStream()
if cheatEngineIs64Bit() then
psize=8
s.Size=16
else
psize=4
s.Size=8
end
if (th==nil) then
writePointerLocal(s.Memory,-2) --currentthreadhandle
else
writePointerLocal(s.Memory,th)
end
writePointerLocal(s.Memory+psize,mask)
if executeCodeLocal("SetAffinityThread", s.Memory)==0 then return nil end
s.destroy()
return true
end
|
_________________
Do not ask me about online cheats. I don't know any and wont help finding them.
Like my help? Join me on Patreon so i can keep helping |
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|